Details
ResourceFunction["HatTrialityTree"] accepts all the options of
Tree, and two additional options:
ColorFunction and "Seeds". Any valid
ColorFunction should accept up to two arguments: the first being a positive integer for vertex "Class", and the second being a bit (either a 0 or 1) describing the "Symmetry" type of the tree to which the vertex belongs. The "Seeds" option accepts a Boolean value,
True or
False, when set to
True, causes
ResourceFunction["HatTrialityTree"] to generate forests rather than isolated trees.
The "Hat triality pattern" is part of a new math discovery following from the groundbreaking research of David Smith et al. It is a three coloring of the hexagonal lattice, which describes valencies of some vertex figures in a Hat tiling.
The Hat tiling is known to be aligned with the hexagonal lattice. The following map is used with lattice vertices at the center of each hexagon:
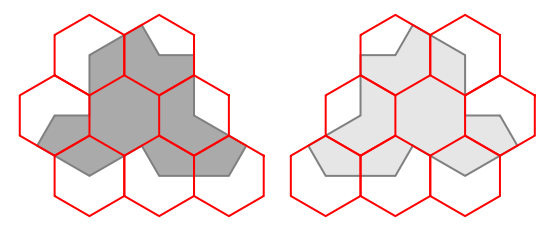
A duality pattern is obtained by classifying interior hexagons and edge-centered hexagons into two separate sets. A triality pattern is obtained from inner/outer duality by allowing edge-centered hexagons to fall into two distinct subsets. Subset membership depends upon how many Hat tiles are incident upon the corresponding lattice vertex, which can be either two or three.
The interior set must consist of isolated vertices so that edge 3-joins link with their neighbors to form ever-larger "triality trees". That is what has been discovered from analyzing large patches of the Hat tiling and the two-dimensional substitution rules that generate them.
The purpose of this function is not to recreate the valency triality pattern, but instead to recreate another more subtle triality pattern, the "mutual trees triality pattern" (see Neat Examples).
However, both "valence" and "mutual trees" triality patterns overlap on the set of edge 3-joins, which are called "the triality trees" or "the triality forest". These trees fall into two subsets. In ResourceFunction["HatTrialityTree"], the two subsets are generated by setting seed to 1 or 2. Unfortunately, any one triality tree is not plane-filling, so it cannot describe the entire Hat tiling.
Closer analysis shows that the root vertices of smaller triality trees can be linked to branches of their older neighbors in a way that covers enough of the hexagonal lattice. When this is done correctly, by setting the option “Seeds”→
True, the Hat tiling can be obtained by mapping H7 and H8 Hat clusters onto leaves of the triality tree (see Neat Examples).
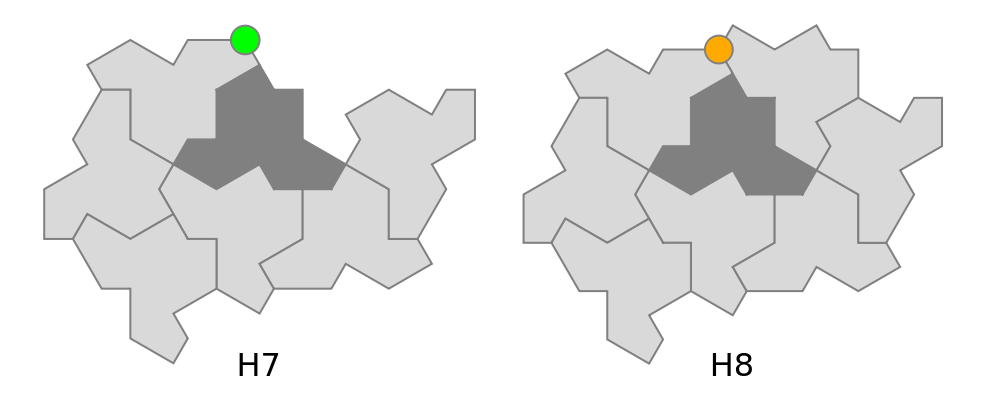
More precisely, reflected Hats at the center of the H7 and H8 clusters are in 1-to-1 correspondence with two particular types of 3-join vertices: proper terminals ("Class"=1 in this data model,
Orange with
ColorFunction→
Hue), and singleton seeds ("Class”=3,
Green). When clusters are drawn differently from the original paper (as above), the minority H7 can be put into 1-to-1 correspondence with singleton seeds.
By mixing some edge 2-joins with all interior vertices, a third tree can be obtained which covers about half the hexagonal lattice by itself, while still fitting in the interstices between isolated triality trees. By setting seed to 0, all types of symmetric trees are generated.
Setting
seed to 3 joins symbols from the symmetric and asymmetric trees, resulting in a growth pattern that must collide on skip vertices ("Class”=0,
LightGray). The resulting trees also only collide on skip vertices. This pattern intersperses symmetric and asymmetric triality trees, leaving a sparse third set of vacancies.